While writing a shape matching app for my daughter I’ve been generating several different shapes. While most were straightforward, creating a heart took a little bit of work and research that I thought I would share to possibly save someone time in the future.
<Canvas>
<Path Stroke="Red" StrokeThickness="3"
Data="M 241,200
A 20,20 0 0 0 200,240
C 210,250 240,270 240,270
C 240,270 260,260 280,240
A 20,20 0 0 0 239,200
" />
</Canvas>
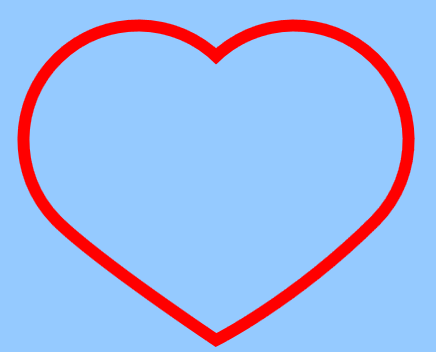
This is making use of 2 arcs and 2 Bezier curves.
M – moves start position to designated location (x, y)
A – specify line size & draw an arc from current to a new position
C – draw cubic Bezier to X, with control points at Y and Z
There are a few different mathematical equations for drawing a heart shape including the following:
x = 16sin^3(t)
y = 13cos(t) - 5cos(2t) - 2cos(3t) - cos(4t)
and
((x^2 + y^2 - 1)^3) - (x^2 * y^3) = 0
Hopefully this saves you some time if you are trying to draw a heart! Good coding!